How to Solve the Crackme Challenge (A Small Reverse Engineering Puzzle)
Welcome back my fellow hackers! Recently, I’ve been delving into the incredibly interesting world of reverse engineering! I hope to write more about this topic some time in the future, but for now we’ll just start with something simple. I took a few days and made a small reverse engineering challenge. Today, we’re going to go through this challenge and solve it with all 3 intended solutions (if you can find more, leave them in the comments!).
We’ll start with downloading and compiling the challenge, then we’ll start solving it. Our first solution will be a buffer overflow vulnerability intentionally coded into the challenge, then we’ll hunt down and execute a secret function to solve the challenge, and finally we’ll execute the program instruction to instruction until we can read the solution straight out of the computers memory! Now that we know what we’ll be doing, let’s get started!
Downloading and Compiling the Challenge
First, we need to download and compile the code of our challenge. Let’s download the challenge code from pastebin using the curl command:
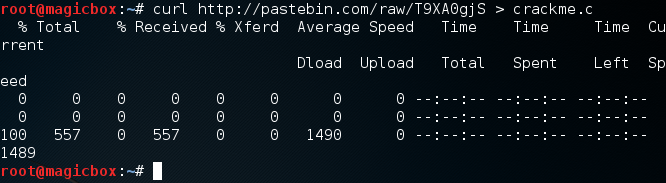
We’ve written the code to a file named crackme.c. Now that we have our code, we need to compile it. We’ll be using the GCC (GNU Compiler Collection) to compile our code. Pay close attention to the flags used when compiling our code, as they are important to the challenge functioning properly:

You may get a warning out of the compiler but don’t fret, the code will still work (this warning is related to the buffer overflow vulnerability, which is intentional). Let’s test our executable just to make sure it works:
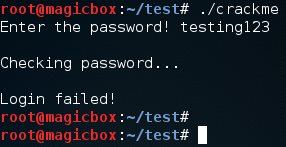
Now that we have our code compiled and working, we can start cracking this challenge! We’ll start with the buffer overflow vulnerability.
Cracking the Challenge
Solution 1: Buffer Overflow
Buffer overflows are very common vulnerabilities. A “buffer” is simply an area of memory designated for storing data to be processed or used in processing. If we can fill a buffer with more data than it can handle, we “overflow” it, and can then store data in areas of memory where we shouldn’t be able to. This can lead to code execution, which is how a lot of buffer overflow exploits work. But, in our case, we’ll be overflowing a buffer simply to change the value of the data being held in another.
Let’s perform this attack, and then we’ll take a look at the code that makes this possible:

We can see here that by entering a large amount of text, we can successfully login even when this is obviously not the correct password. Now that we’ve exploited our buffer overflow, let’s take a look at the code that made this happen:

We can see here that we make a character array that holds 30 characters. So, if we input more than 30 characters, that will overflow this buffer, which will change the value of the “valid” integer. This integer is then evaluated later in the program and, since the overflow changed it to a non-zero number, it equates to true and logs in without a problem. Next up, we’ll be hunting for a hidden function in our program!
Solution 2: Using the Hidden Function
If you read the code of our challenge, you’ll that there is a function named “secret.” This function is never used, yet it still exists. If we can force this function to execute somehow, we could get the password we need. Well we have just the tools to do that! First, we need to use a tool called objdump (object dump) to disassemble our executable into assembly. Then we’ll be able to see where our secret function is:

Now that we have our new assembly code in a text file, we can sift through it until we find our secret function. It should be just above the main function, and looks something like this:
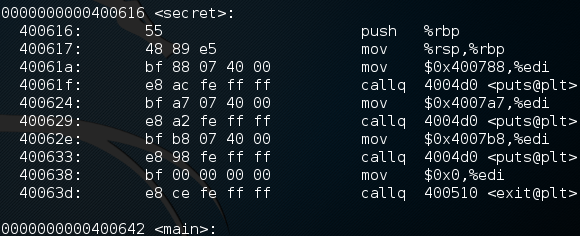
Now, don’t panic, this looks way more complicated than it is. The only part of this assembly we need to pay attention to is the string of characters next to the word “secret.” Specifically, we need to remember the last 7 characters, as this is the entry address for this function. Now that we have this 7 character string, let’s start our program in GDB and do some magic:

No need to pay attention to this large chunk of text, it’s mostly just information about GDB. But here’s the important part: when GDB starts up, we need to use the start command in order to start our program. Now, remember that 7 character string? This is where we’ll be using it. If we can change where the instruction pointer is pointing, we can force it to execute our secret function. Luckily for us, GDB allows us to do just that:
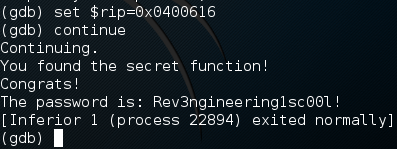
We need to precede our string with a “0x” as this specifies it as a memory address. Now that we’ve wrapped up this solution, on to the next, reading the password out of memory!
Solution 3: Reading the Password out of Memory
SIDE NOTE: For this solution to work you may want to re-compile the program with the -m32 flag to compile a 32-bit version of it.
Our final solution is the most complicated. When we enter a password into the program, it needs to be stored in memory so it can be evaluated later. If we can catch it while it’s stored in memory, we can read it! We can also use GDB for this. So, let’s start up GDB again:
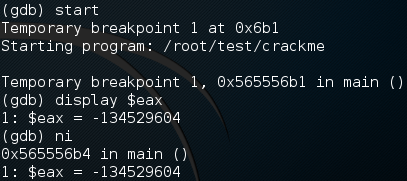
I’ve cut out the large chunk of text shown by GDB for the sake of organization. Now, we need to start the program with the start command. Once we do, we display current number of the EAX register. This is the register that our password will be in once we enter it. After we display the current contents of EAX, we use the ni command (next instruction) to move on to the next instruction in the program.
As you can see by the output above, once we used the ni command, it displayed the value of EAX again. We can use this to tell when the value in EAX changes, if the number changes, the contents changed. Now we just need to repeatedly press return to re-execute the ni command and step through our program. Once we reach the prompt for a password, enter something random:
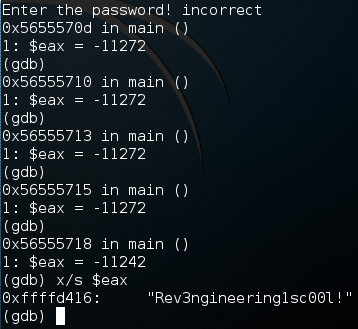
We can see that a few instructions after we entered an incorrect password, the value of EAX changed. Now we can use x/s to read the contents of EAX as a string which, in this case, is the correct password to our program!
This concludes our small reverse engineering article. I’d like write more about this in the future, but since I’m still learning myself, we’ll have to save it for another time. Just know that this idea is on the back-burner for now!
Buffer Overflow explained through C code
Buffer Overflow explained through C code
Buffer overflow is a well known vulnerability . It is one of the most frequent attack types.
It uses input to a poorly implemented, but (in intention) completely harmless application, typically with root / administrator privileges. It results from input that is longer than the implementer intended. To understand its inner workings, we need to talk a little bit about how computers use memory.
Buffer Overflow through C language .
Lets take an example C program that has a this vulnerability . The vulnerability doesnt exist in the C language or the compiler but it exists in the strcpy function . This function is vulnerable to buffer overflow as it doesn’t check for the memory bounds of the data it copies .
Let study some real program examples that show the danger of such situations based on the C. In the examples,I have not implement any malicious code injection but just to show that the buffer can be overflow. Modern compilers normally provide overflow checking option during the compile/link time but during the run time it is quite difficult to check this problem without any extra protection mechanism such as using exception handling.
C code to Show Buffer overflow
#include <stdio.h> #include <string.h> #include <stdlib.h> //Author : Vanshit Malhotra //Demonstrate Buffer Overflow via Segmantation Fault int main(int argc, char *argv[]) { char mybuffer[5]; if (argc < 2) { printf("strcpy() NOT executed....\n"); printf("Syntax: %s <characters>\n", argv[0]); exit(0); } strcpy(mybuffer, argv[1]); printf("mybuffer content= %s\n", mybuffer); return 0; }
Here are the screenshots from the execution of the code .


Observe the SEGMENTATION FAULT that occurs when data more than the buffer limit is provided as an input to the program .
You might like using strcpy_s() instaed of strcpy() function to avoid buffer overflow in your C code .
The vulnerability exists because the mybuffer could be overflowed if the user input (argv[1]) bigger than 8 bytes. Why 8 bytes? For 32 bit (4 bytes) system, we must fill up a double word (32 bits) memory. Character (char) size is 1 byte, so if we request buffer with 5 bytes, the system will allocate 2 double words (8 bytes). That is why when you input more than 8 bytes; the mybuffer will be over flowed .
Similar standard functions that are technically less vulnerable, such as strncpy(), strncat(), and memcpy(), do exist. But the problem with these functions is that it is the programmer responsibility to assert the size of the buffer, not the compiler.
BAD USB Attack : Hacking by Just USB
BAD USB Attack : Turn the USB into Hack Tool
BAD USB Attack executes the malware without any interaction of the Victim (opening the USB or double click on any file not required) .
USB these days have become the prime source of Data transfer . The USB devices are very Ubiquitous , and have been used by everyone .
The Bad USB is an attack where the USB infects the machine without the user even noticing . This attack makes it possible to infect machines with malware and easily spread the malware , and that to undetectable by the user as there is no interaction of user required .
This attack requires the hacker to reprogram the controller chip in the USB peripherals . Very widely spread USB controller chips, including those in thumb drives, have no protection from such reprogramming.
BAD USB : Turning the USB into Evil Devices
Once the USB Controller Chip has been Reprogrammed , the USB can be used to spreading the Malware undetected . The Bad USB attack can act evil in one of the following ways :
[box ENGINE=”shadow” align=”” class=”” width=””]
- A device can emulate a keyboard and issue commands on behalf of the logged-in user, for example to exfiltrate files or install malware. Such malware, in turn, can infect the controller chips of other USB devices connected to the computer.
- The device can also spoof a network card and change the computer’s DNS setting to redirect traffic.
- A modified thumb drive or external hard disk can – when it detects that the computer is starting up – boot a small virus, which infects the computer’s operating system prior to boot.
[/box]
BAD USB Source Code has been released . Here is the Github link .
Protection From BAD USB Attack :
No Effective Defences Known yet .
Malware Scanners cannot access the firmware where the malware actually resides in the BAD USB Attack . Behavioural detection is difficult since behaviour of an infected device may look as though a user has simply plugged in a new device.
Also the BAD USB Attack is hard not only to detect but also to clean Up . The Clean up of the malware from the Victim Machine post attack is very difficult to make the matters even worse . Reinstalling the Operating system is the standard response here .
Also to clean-up the USB , reinstalling the firmware is mandatory .
Hacking Hackers Phish Pages to View Hacked Facebook and Email accounts using Google
Hacking Hackers Phish Pages to View Hacked Facebook and Email accounts using Google : Welcome friends, today i am going to teach you how to see the hackers illegal phish pages password file using Google, which all the passwords of victims are stored. As we all know 99 % hackers over internet are novice hackers or simply script kiddies. They don’t know the concepts and they just follow or use the material available on internet to create Phishers to Hack Facebook, Gmail or simply passwords. Which always result in creating Phisher successfully but not a secure one because none tells the after phisher technique like how to make phishers undetectable, how to protect password files where all hacked passwords are stored etc. As most of us know that Phishing is the easiest method over internet to hack Facebook and Email account passwords, so most novice hackers opt this option to hack victims passwords. In fact some professional hackers uses Phishing technique too but they are bit advanced and prefer tabnabbing(click here to learn more) over Phishing. As Hackingloops concentrates on concepts and digging the root causes. We will learn something better that no body does or tells i.e. how to hack the hackers. What loophole we are digging today?? Any idea?? No ?? So go on.
![]() |
Hacking Hackers Phish Pages to View Hacked Facebook and Email accounts using Google |
Loophole
Most of us who are webmasters i.e. people who design websites knows the concept of Google indexing but others might not have that good idea. So let me explain first. How any website results appears in Google search results? You made the website and how does Google knows you website? All search engines uses spider and crawler software’s over the web to index the new websites or latest changes in the existing websites in order to give users the best latest results. And indexing of website depends on a file located at root level of all web hosting websites, if its not present Google treats as full index. Most people think that robots.txt file is used to tell Google to index your website but actually robots.txt file used to tell Google that what you want to index from your website and what you don’t want. By default, robot file allow full website indexing i.e. all files are indexed even password and database files. Woooo! Here the loophole lies. Most almost all hackers uses free web hosting websites to run the Phish pages and all free web hosting websites have default robot.txt file which means when hacker uploads its phish pages, its indexed by Google. And we all know what we use to extract smart information from Google? Off course its Google Dorks. Now we have to learn how to make our own dorks to extract hackers phish page information.
Hackers edits Form redirects of any login page and change the request mode from POST to GET in order to retrieve passwords in plain text and then they stores it in simple text and html files. Today we are going to learn how to extract those password files using Google. Well there is not much to learn in it because i have already made the DORK for you, what you have to do is just enter the same in Google and you will have access to all Hackers Phish pages password files containing all the hacked password they hacked till now.
No comments:
Post a Comment